Understanding Python messages
Your first and most helpful debugging tool is the interpreter itself. When something goes wrong, it will tell you what went wrong, and try to identify the line where it went wrong.
To see this in action, download and run this file at the command prompt: test1.py. You should see the following:
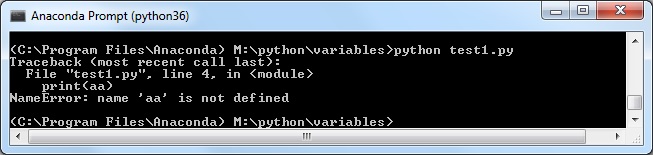
There are several pieces of information here. Firstly Traceback (most recent call last):
. This
reminds you that if your problem causes several issues, the one you probably want to look at is
the last one, as that's the thing that tried to run and didn't work. All the other issues above it resulted
from that problem.
This is the situation with one bug, which can generate multiple issues. It is also try that you can have several bugs, each causing one or more issues – Python may list more than one of them, but often it will just list the first it hits, coming back with additional issues as you fix those earlier on in the program. The easiest thing is always to start at the bottom of the list of issues and fix the issue. If there's only one bug, the issues will all disappear. If there is more that one bug, the new one will appear at the bottom of the list.
Secondly, the message tells you the line(s) where it went wrong and prints the line. Here we've just got one, but, in reference to the Traceback message, there might be several and you usually want the last.
File 'test1.py', line 4, in <module>
print(aa)
Here it tells us that the issue is in line 4 of test1.py. If our code was in a module (a library of code), it would also give the name of that.
Lastly, it tells you what the problem is likely to be:
NameError: name 'aa' is not defined
This is a classic error. It means we're trying to use something, but the system doesn't know
what it is; in this case, aa
. If you look at the code, you'll see that we've mis-typed
the variable name we're printing:
a = 0
print(aa)
It should be:
a = 0
print(a)
Fix the code and then rerun it to check it works. Once you're happy with it, go on to look at different issues with variables.
- This page
- Issues with variables <-- next
- Practice
- Answers