Communication
In this practical, we're going to get our agents talking to each other by altering each other's variables.
In order to do this, several things have to be in place.
Firstly, all our agents need to know about each other, so they all need the list of agents.
Secondly, we need them to have a method they can call upon in order to check their neighbourhood.
Finally, we need them to scan the list of other agents, and if they find any within a neighbourhood of themselves,
we need them to adjust their variables and their neighbours' variables in order to transfer information to the
other agents. Here's the final UML:
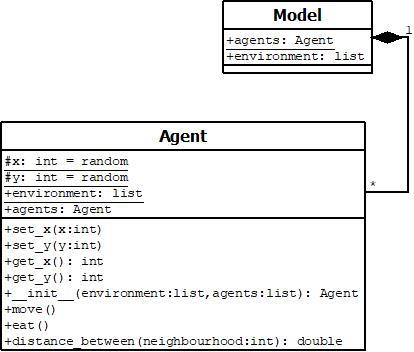
Let's start by getting a link to the list of agents into each agent. Given what we did in the last practical to get the environment into each agent, can you get the list of agents into each agent?
Note that the list doesn't need to be full of agents when you pass it into each agent: the link is to the list, not a copy of the list; provided it is full of agents before you use it, it can be empty or half full when you pass it into each agent.
Get this into your agent, and print another agent's y
or x
to prove it.
Next, we're going to start constructing another behavioural method for our agents. At the moment, we have move
and eat
. We're going to
make a function called share_with_neighbours
in which the agent will search for close neighbours, and share resources with them. First, let's write
the method call. In model.py
, add a new variable towards the top of your code, neighbourhood
, and set it to, say, 20. You should have
something like this at the top of model.py
:
num_of_agents = 100
num_of_iterations = 10
neighbourhood = 20
Note how we're collecting all of the model parameters that we might want to use to adjust the model towards the top of the model. This is good practice: it means we don't have to search around for them if we want to adjust the model. At the end of the practical we'll have some suggestions for using this.
Adjust the looping through agents to:
for j in range(num_of_iterations):
for i in range(num_of_agents):
agents[i].move()
agents[i].eat()
agents[i].share_with_neighbours(neighbourhood)
And write an appropriate method declaration in Agent to run when this call happens. Get it to print out the neighbourhood
passed in to check it works.
We'll now go on to build this method.