Classes and objects
Having tested the Agent code on one agent, you should now be in a position to adjust the model.py
to look something like this:
import random
import operator
import matplotlib.pyplot
import agentframework
def distance_between(agents_row_a, agents_row_b):
return (((agents_row_a.x - agents_row_b.x)**2) +
((agents_row_a.y - agents_row_b.y)**2))**0.5
num_of_agents = 10
num_of_iterations = 100
agents = []
# Make the agents.
for i in range(num_of_agents):
agents.append(agentframework.Agent())
# Move the agents.
for j in range(num_of_iterations):
for i in range(num_of_agents):
agents[i].move()
matplotlib.pyplot.xlim(0, 99)
matplotlib.pyplot.ylim(0, 99)
for i in range(num_of_agents):
matplotlib.pyplot.scatter(agents[i].x, agents[i].y)
matplotlib.pyplot.show()
for agents_row_a in agents:
for agents_row_b in agents:
distance = distance_between(agents_row_a, agents_row_b)
Give it a go. You'll see it's a lot cleaner and easier to understand than before. The model clearly makes some agents and moves them around, and the code in the Agent class is clearly structured in a way that makes it much clearer what all the components are.
That's it for the moment. If you have some time, see if you can do the decent object oriented thing, and protect the self.x
and self.y
variables
by renaming them self._x
and self._y
and implementing a property
attribute for them, with the
appropriate set and get methods: details in the Property documentation. Once you've done this, compare your
code with the UML shown earlier:
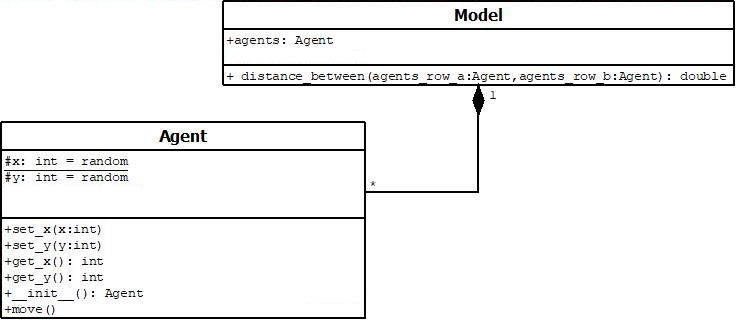