Methods II
[Framework practical 4 of 7]
Next, the method to save our data into a 1D array. This will be built in the Storage class.
Images, strangely, are stored in 1D arrays. This is because 1D arrays are slightly more efficient to process. The data inside an image array is actually stored in quite a complicated format, as we'll see in our lecture on images. However, for now, let's just copy the data out of our 2D array and into a 1D array of the same, double, type.
First up, declare a method in Storage with the following characteristics:
Return type: double[]
Name: get1DArray
Parameter variable: none
Second, the array conversion.
The first thing we need to do is decide which array we're going to convert to 1D. We could use data
, however, we want to
use this method in a sequence to make an image. Given this, we want to get hold of a reranged array. Our first line in our get1DArray method
therefore needs to be:
double[][] reranged = getRerangedData(0.0,255.0);
Note that here we're calling another method in our Storage class from within another method. This means
that we don't need to call store.getRerangedData(0.0,255.0)
-- we are *inside* the store object; we can just
call the method directly.
Next, we need to convert the 2D array into a 1D array. The way we do this is a bit like laying each row end-on-end to build up the 1D array. Here's the algorithm:
// Make a double tempArray [reranged.length * reranged[0].length]
// Open loop with index i down reranged rows
// Open loop with index j across a row
// tempArray[????] = reranged[i][j]
// End loop across row
// End loop across
// return tempArray
The question is, what is the position in a 1D array (tempArray[????]
) that is correct, given our position within our 2D array
(reranged[i][j]
). That is, where in the 1D array is it that we want to copy the 2D data to?
Can you work it out? Note that we'll need to use the nested loop structure indices to navigate across the 1D array as we travel across the 2D array. The values you have to work with are:
reranged.length // The total number of rows
reranged[0].length // The total number of values in a row
i // The number of rows moved down, starting at row zero
j // The number of values moved across the current row
Note that this uses reranged[0].length
rather than reranged[i].length
as positioning would be much more complex if the
second dimension length were to change -- you'd need a different algorithm.
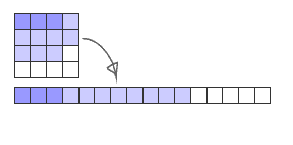
If it helps, try drawing a 2x3 and a 1x6 array and working through it by hand. If you struggle, the answer is here, but try and work it out first!
Again, once you think you've got it working, test that the method works ok from the Analyst class.
Once you've finished that, if you want more practice, feel free to build any other methods you think might use useful or
interesting, especially if you didn't in the last practical (a setDataCell
or clearData
method, for example). We've also
uploaded more test pieces to the Practice Pieces page, and there are some ideas for code to help you understand more on the lecture
note pages. In addition, at some point, please read through the links on UML on the
Useful Links page.
Once you're happy with the code, you are done for this practical.